Firebase
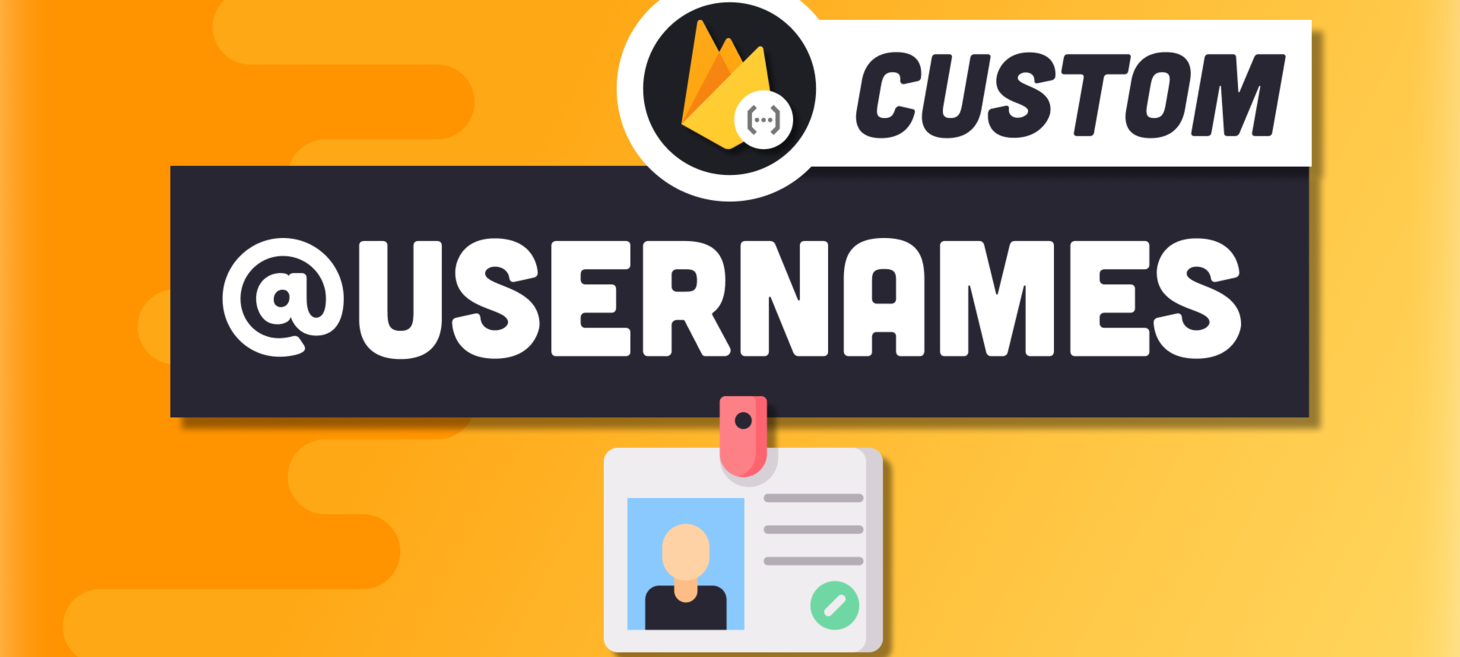
How to implement unique usernames with Firestore
Some apps allow their users to choose their preferred username. This typically happens during the onboarding flow, and allows people to establish their personal brand across apps and platforms.
This Fireship article shows an easy way to implement this, completely based on Firestore, without requiring Cloud Functions or any third-party frameworks.
The article (and accompanying video) are written for JavaScript, but the principles are independent of the implementation language.
I'd also like to point out that this article includes a great example for Firebase Security Rules. You do use Security Rules to keep your Firebase apps secure, right? Right?!
Swift
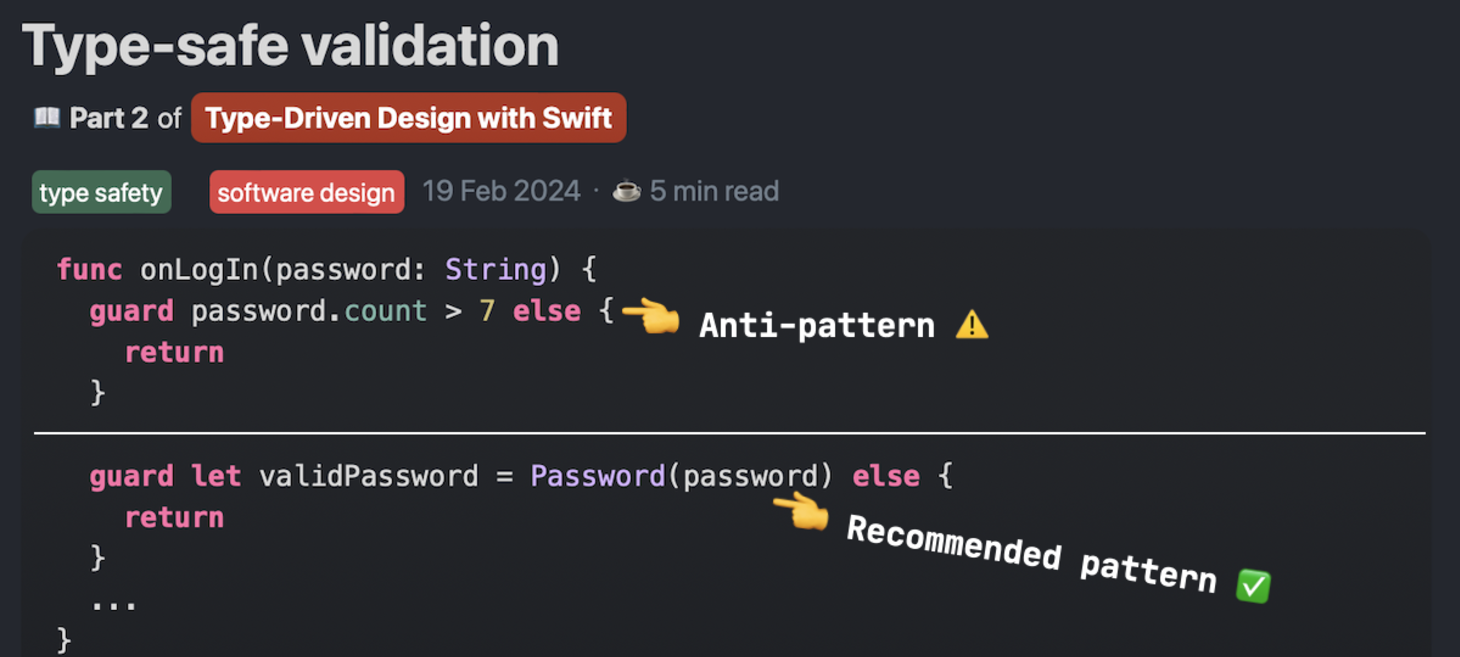
Type-safe validation
I'm pretty sure you've implemented email / password validation at least once or twice in your life as a software developer.
What if I told you that you've probably done it wrong all of this time? Oh, and probably not just email / password validation, but all other sorts of validation logic as well. Spoiler: it has to do with the locality of the validation logic, and loss of knowledge.
Read this article to find out why, and learn how to do better. It's part two of an entire series of articles about Type-Driven Design with Swift by Alex Ozun, and I can't wait to read all the other articles in the series.
SwiftUI
LabeledContent in SwiftUI
LabeledContent
definitely is one of the most under appreciated views in SwiftUI. I knew about its existence, but learned from Danijela's article that you can even stack labels - check it out!
AI and ML
Keyframer: Empowering Animation Design using Large Language Models
Apple is researching how to use LLMs to use natural language to animate designs. In this paper, Apple researchers describe how they used LLMs to allow users to create CSS animations for static SVG images.
Maybe this will one day be part of Keynote?
Until such a time, come to my session "One more thing - How to create insanely great slides using Keynote", in which I show you how to create amazing presentations using Keynote.
Coming to a conference near you, for example SwiftCraft or SwiftHeroes.
Create a Murder Mystery game powered by Gemini AI with SwiftUI
You can use AI for all sorts of tasks - generating or summarising text, creating images and videos, etc. One category of tasks that I find particularly interesting is using LLMs (large language models) as an execution engine for an algorithm that you specify in natural language.
Here is a fun example of a a Murder Mystery game implemented using Gemini and SwiftUI. In his article, Anup D’Souza mentions that the model responses don't match the expected JSON format. I found that this is something you can work around by specifying the exact format you expect.
Here is part of a prompt that I use in a similar game:
Act as a text adventure game in the style of the Infogrames text advemtures.
Provide the result in two blocks: the first block MUST ONLY contain the description, formatted as Markdown.
After the description, insert a newline, and a divider of exaclty FIVE dashes (like this: "-----"), followed by the following JSON structure:
{
description: String,
health: Int,
location: String,
inventory: [String]
commands: [String]
}
This way of prompting ensures the model returns valid JSON.
Security
An introduction to passkeys: what they are, and how they work
We all know that passwords are bad - it's hard to come up with a good memorable password (and quite frankly, your passwords should NOT be memorable), and that is why most users still (!) reuse their passwords.
Passkeys are an approach that was created by an industry consortium to create an alternative that is safe, secure, and easy to use.
You probably heard about passkeys before, and might have wondered what they are. In this article, Bruno explains why they were invented, how they work, and what some of the downsides are.
If you'd like to dig deeper after reading this article, I recommend this WWDC presentation: Move beyond passwords
Design
Creating custom SF Symbols
There are more than 5000 SF Symbols, but if none these match your specific use case, here is an easy tip by Axel Le Pennec to create your own custom symbols.
When I last wrote about this in issue 27 of Not only Swift, SF Symbols had "only" 4500 symbols... Time flies!
If you'd like to learn more about SF Symbols, check out this article by David Smith :
Fun stuff
Bored at work?
You probably knew about the Chrome dino that helps you pass time as you wait for your internet connection to come back on, but did you know there is a text adventure in Chrome?
These past two weeks, my Twitter feed was full of controversy around Xcode - some people love it, some hate it. I have to admit that I fall into the camp of people who love it - although there is a bunch of things I'd love to see improved, and don't get me started on (the lack of) support for refactoring.
What are the features you miss most in Xcode, and what is something you think it does better than other IDEs?
Don't forget to send in your hidden gems - videos, blog posts, documentation, or tweets that you came across and which you feel didn't get the attention they deserve. You can submit them via this form: bit.ly/not-only-swift-submission).
Thanks for reading!
Peter