Firebase
Sign in with Apple Updates
We've recently launched support for filling the user's displayName
when signing in with Apple. You can now call the following API - no more need to update the displayName
using createProfileChangeRequest
.
let credential = OAuthProvider.appleCredential(
withIDToken: idTokenString,
rawNonce: nonce,
fullName: appleIDCredential.fullName)
We've also launched support for revoking a user's access and refresh tokens when deleting their user account - which is a requirement for all apps that offer account creation.
The steps for deleting a Firebase user account who has signed in with Apple are:
- Ask the user to authorize deleting their account. This is required to get an authorisation code, which Firebase needs to revoke the user's tokens.
- Call the
Auth.auth().revokeToken(withAuthorizationCode: authCodeString)
to actually revoke the token - Once that's succeeded, you can delete the Firebase user by calling
user.delete()
- To also delete their associated data, I recommend using the Delete User Data Firebase Extension
You can find more details in the documentation for Signing in with Apple with Firebase. As mentioned in the last issue of the newsletter, I've recorded an entire video to walk you through deleting user accounts (including token revocation), which should be published soon - so why don't you subscribe to the Firebase YouTube channel to make sure you don't miss it!
Swift
Swift Community Awards
The Swift Community Awards have been nominated - congratulations to all winners, and thanks to everyone who cast their vote!
Abusing Swift’s Result Type. A tale about when the Result type…
When it was added to Swift, Result
solved a real pain point in API design - completion handlers that return either a result, or an error - or maybe both?
From looking at this signature, it is entirely unclear which behaviour to expect:
func getData(_ completionHandler: (_ data: Data?, _ error: Error?) -> Void)
Nikita takes us on a trip down memory lane, and ends with a plea to do the right thing and... but see for yourself.
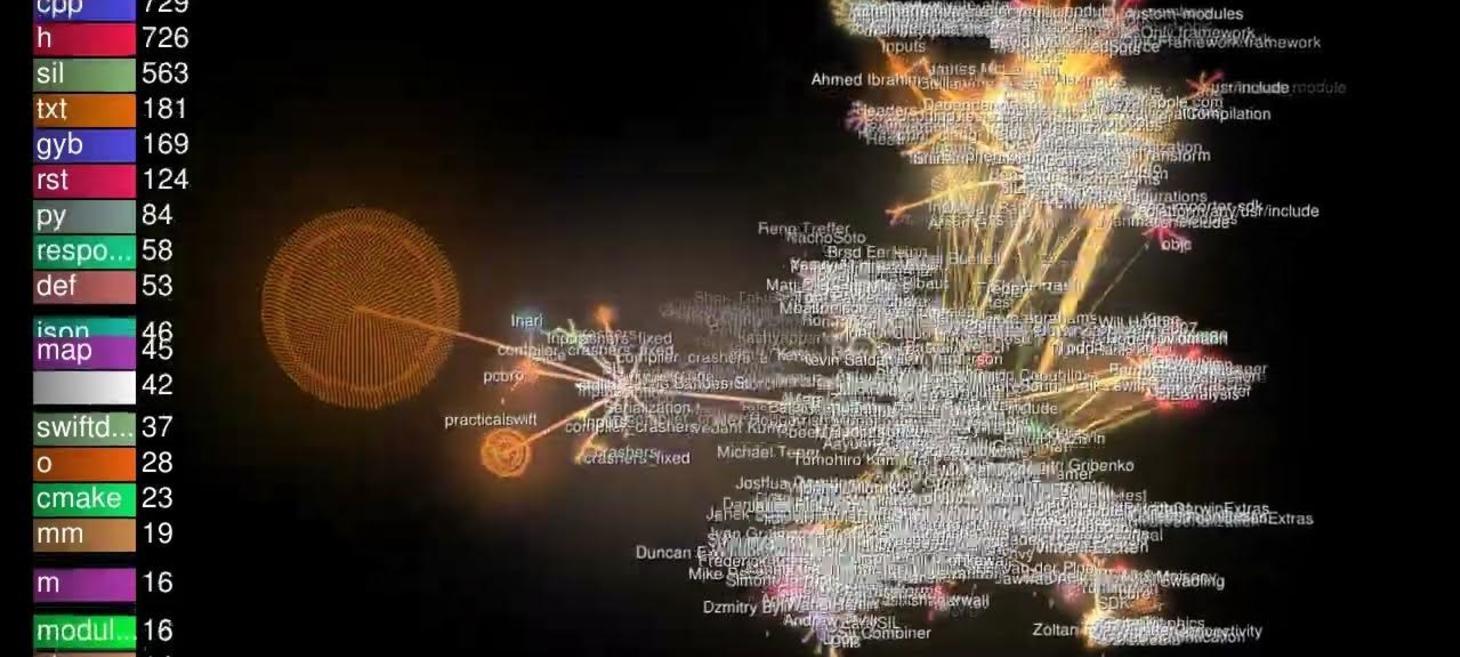
The Swift Language Visualized with Gource
This is a really impressive visualisation of the Swift repo using Gource.
I love how the cluster for the compiler flashes up every now and then - it looks like someone is beaming a ray of energy into it.
Preparing my app for Swift 6
In issue #35, I mentioned piecemeal adoption of upcoming language features for the first time, and this week, Cihat wrote a blog post about how he prepared his app for Swift 6 with this feature.
It's a pretty interesting read, and it seems like it was a smooth experience. If you want to get a head start for Swift 6, read this article to learn how.
SwiftUI
Deeplink URL handling in SwiftUI
Antoine walks us through setting up deep links, capturing them with SwiftUI's onOpenURL
view modifier, and discusses some interesting use cases.
With great power come great responsibilities, and Antoine discusses these in the security implications. Definitely worth a read!
The Ultimate Guide to the SwiftUI 2 Application Life Cycle
Deep linking is only one aspect of application start-up that has changed when Apple launched the new SwiftUI life cycle at WWDC 2020. For more details about detecting your app's foreground / background state, continuing user activities, and handling deep links, check out The Ultimate Guide to the SwiftUI 2 Application Life Cycle
Tools
Interface Builder for SwiftUI
Marcin shared a new feature in Judo: creating SwiftUI code from your designs in realtime.
This reminded a bit of PaintCode. While Judo seems to mostly target designers who are looking for an easier way to hand off their designs to developers, I seem to recall PaintCode was made for developers who were looking for a way to turn vector drawings into code.
I wonder if the Xcode might have an improved version of the SwiftUI preview canvas up their sleeves.
If you'd like to give Judo a try, there is a TestFlight link in this tweet.
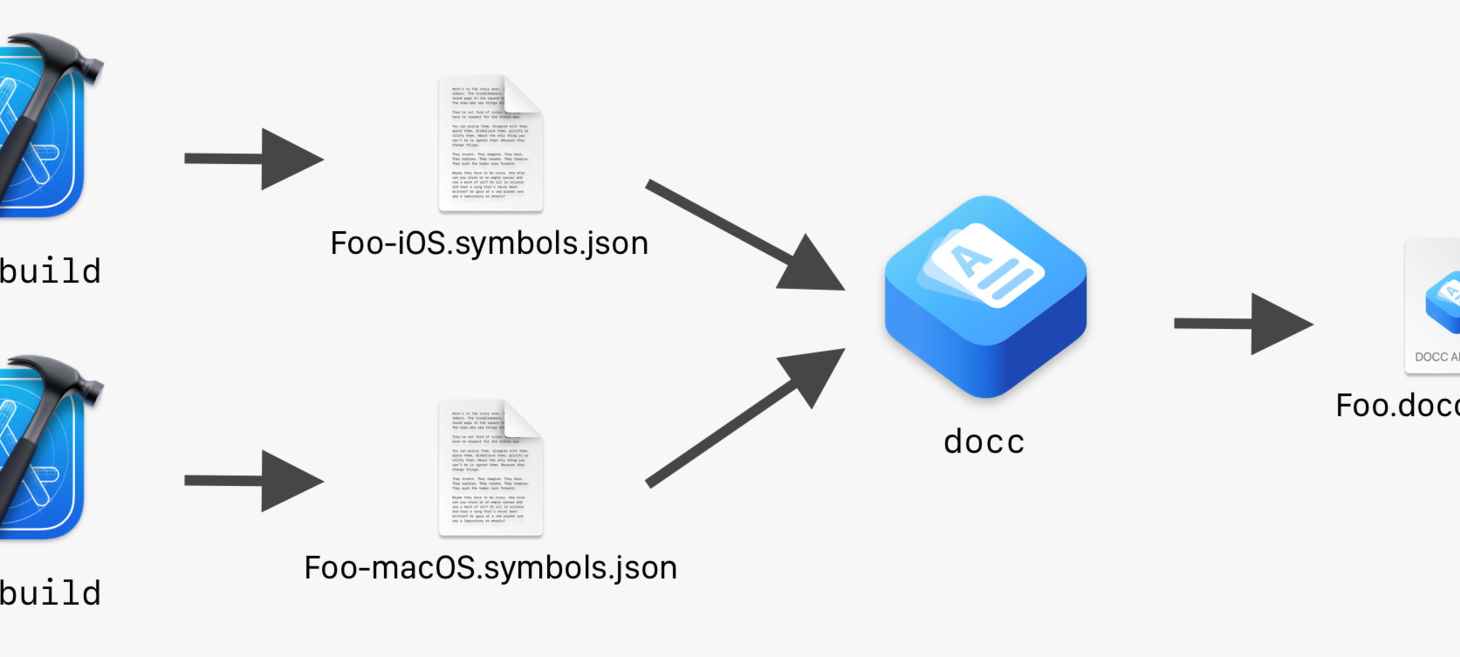
Building Documentation for Both iOS and macOS Using DocC
DocC is a fantastic tool for generating reference documentation and interactive tutorials for your framework or package.
If you're building a package that supports multiple target platforms, some of your API might be platform-specific, and your documentation should reflect that.
Simon describes how you can set up a DocC build script that takes this into consideration.
Authentication
Skip typing your login credentials manually with Xcode breakpoints
Bringing an app into the initial state for testing a specific feature or reproducing a bug can be a tedious process, especially when having to go through a login process. Danijela discusses an interesting approach that makes use of Xcode breakpoints. Be aware though - this requires exposing the respective password in a breakpoint expression.
If you'd rather sign in without having to type in a password, consider implementing Anonymous Authentication, or use read Antoine's article about deep links in the SwiftUI section of this issue of the newsletter.
Contrary to popular belief, working in Developer Relations doesn't mean you're speaking at events and traveling the world all of the time. A large chunk of the work is doing the grunt work to improve the product(s) you stand for, and make them better for the people who use them.
This week marks the culmination of a whole bunch of work that I've been busy with for the past couple of months, and I am super proud of what we've achieved. On the face of it, adding support for token revocation for Sign in with Apple and filling the display name of the Firebase user when signing in sound like tiny features, but both involved a lot of work behind the scenes: advocating for the users and highlighting the pain they had to go through without those features, convincing teams to prioritise the work, writing code that demonstrates the issue, discussing possible solutions, implementing and testing them, throwing them away when we realised they didn't work, writing test cases, going through security reviews, updating the docs, keeping people updated on the GitHub repo and on Twitter, scripting and recording videos, and - finally - flipping the launch bit and pushing the submit button.
So - whenever you get the impression things aren't moving forward as quickly as you'd like them to - keep in mind that you only see the tip of the iceberg. But also, don't be shy to reach out to your friendly neighbourhood Developer Relations person to ask them for an update - that's what we're here for, after all!
Thanks for being a subscriber - it means a lot to me!
Peter ❤️🔥